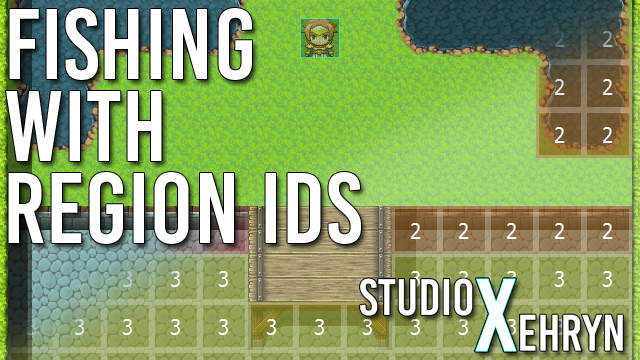
Fishing with Region IDs
Implement one aspect of a fishing system using common events and region IDs.
Engine: RPG Maker MV / MZ
Difficulty: Easy
Introduction
When it comes to fishing systems, there are two aspects to consider: the fishing spot, and the fishing minigame. This tutorial will cover the fishing spot, as I have already made a tutorial on a fishing minigame, seen here (reflex / timed press minigame)! Please note: that tutorial uses VX Ace, but the method can be easily ported to MV / MZ!
This system for determining the fishing spot will lets the player fish anywhere that is marked by region IDs. The other tutorial also covered fishing spots, however the way it is done is restrictive and requires making a lot of duplicate events, which can introduce lag! With this system, we can make any body of water fishable, or even have a body of water contain multiple spots, like in Pokemon. In fact, it doesn't even have to be water, just as long as it's marked with a region ID!
Because this system is evented, it is possible to use this in other RPG Maker versions, with some modifications to script calls.
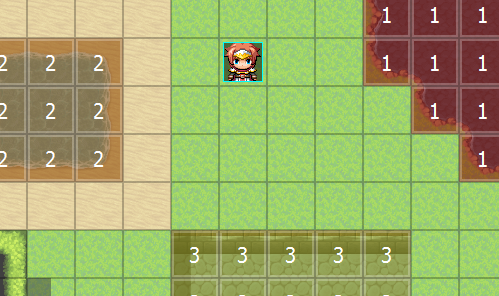
Each pond here can give a different fish, and we can fish at any tile without the need for a hotspot! You can even mix the regions together!
Why This Approach
This method has a number of advantages over using events hotspots:
- You can line the entire edge of a body of water without worrying about lag from too many events.
- Avoids having hard-to-see event hotspots, as some events might blend in too well.
- You can change the system without having to modify possibly hundreds of events across maps due to everything being in one common event vs spread out throughout your game.
- You can have bodies of water that have location-dependent fish, such having different fish in the center of a lake vs on the edge.
The Gist
We'll be setting up a common event named "Determine Fish" that is called by the Fishing Rod item when used. "Determine Fish" will then check the tile directly in front of the player for a region ID, and we will use that region ID to determine what fish the player would receive.
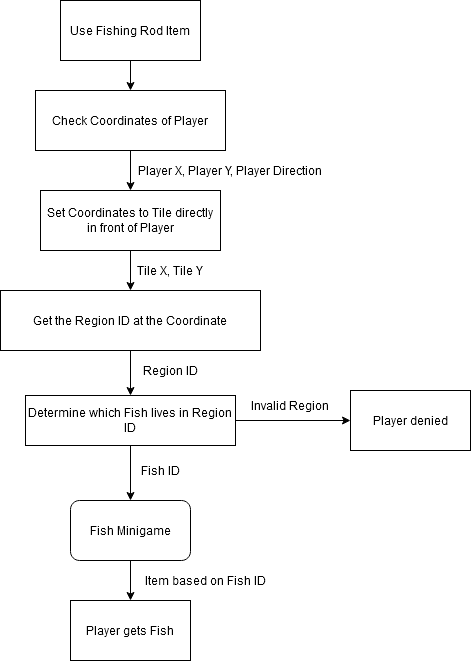
Each arrow in the diagram represents data passed down to the next step. (...The diagram ended up kinda looking like a fishbone?!)
What We Will Need
- Fully Evented - 4 Variables: Player X, Player Y, Region ID, Fish ID
- With Script Calls - 2 Variables: Region ID and Fish ID
- 1 Common Event: Determine Fish
Setting up the Database
In the items tab of the Database, create your items for the fishing rod and the fish. The Fishing Rod item should be a Key Item that can be used repeatedly. Remember to set Consumable to No, Scope to None, Occasion to Always.
The Common Event - Getting the Region ID
For the common event, we'll first get the player's coordinates on the map. Then, we'll check the player's direction and adjust the coordinates to move one tile in the player's direction. Using these coordinates, we will check the region ID and set it to a variable. (Pictures below.)
To get the player's coordinates, set two variables to the player's map X and Y. With events, use Control Variables -> Game Data -> Character -> Player -> Map X.
Now, check the player's direction with Conditional Branch, page 3. Nest these conditional branches with an Else branch and check the other directions. For Down, add 1 to Y; Up, subtract 1 from Y; Left, subtract 1 from X; Right, add 1 to X.
Now we have the coordinates of the tile in front of the player. Use it by going to Event Commands page 3, Get Location Info. Set the variable to a new variable, Region ID, set the Info Type to Region ID, and set Location to Designation with variables, with our X and Y variables.
Your common event will look something like this:
That takes up quite a lot of space, so if you'd like, you can make it using a single script call (variable 3 is Region ID here):
x = $gamePlayer.x;
y = $gamePlayer.y;
switch($gamePlayer.direction()){
case 2: y += 1; break;
case 4: x -= 1; break;
case 6: x += 1; break;
case 8: y -= 1; break;
}
region = $gameMap.regionId(x,y);
$gameVariables.setValue(3, region);
The Common Event - Determining the Fish
Now that we have the Region ID, we will check what fish will appear. But first, we need to make sure to handle what happens if there is no region ID; if the player uses the rod somewhere without water. Set up a check for Region ID 0, which is if there is no ID marked. You'll want to Exit Event Processing if the Region ID is 0, so the player can't fish where they aren't supposed to.
Now for determining fish for valid Region IDs. This is as simple as just using a conditional branch to check the Region ID number, and setting a variable Fish ID to whatever fish we want to appear for that region. Note that Fish ID isn't the same as an item ID in the database! It's simply a way to keep track of types of fish in your game, and it can be whatever you want. You may choose the item ID as the Fish ID if it makes things easier.
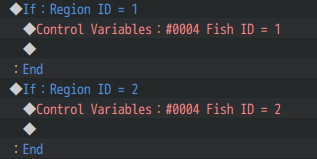
You can copy and paste your conditional branches. Keep in mind that regions go up to 255 - though I don't recommend having that many variations or fish!
Maybe you want multiple fish in the same region ID, and have it be random which fish is found? You can do that with a script call and an array, like so (variable 4 is Fish ID here):
fish = [1, 2, 5, 8, 10];
fid = fish[Math.floor(Math.random() * fish.length)];
$gameVariables.setValue(4, fid);
Put all the Fish ID in between the square brackets, separated by commas. You can increase the chance of a fish being picked by adding it multiple times, like [1, 1, 1, 2, 5, 8, 10].
The Common Event - Starting the Fishing Minigame
After determining which fish the player will find, call the common event for the fishing minigame (not included here, click here for that tutorial). Of course, if you don't want a minigame and just want to give the fish directly, just jump to the section "Part 3: Rewards".
Final Result
Here's how the common event looks after it is complete. Of course it'll look different for your game, as there'll be plenty of fish to catch!
Setting the Regions
Now that you have the common event done and the usable Fishing Rod that can call the common event, you will need to make it possible to fish on the map! Here's a quick example.
On the tileset palette, click on the R tab to show regions. You can then paint regions like any other tile. Region 0 is the blank region.
In this example, the western pond will give us Fish ID 1, the eastern pond will give Fish ID 2. However, the southern pond has three different region IDs! On the west side of the bridge, along the shoreline, we'll find Fish ID 1, and on the east Fish ID 2. But if we go onto the bridge, we can find five different Fish IDs! If your game gives players the ability to travel on the water, you can take better advantage of the inner sections of water.
Be mindful of other plugins that use regions. You will need to add those regions to the region-check part of the common event so the game knows it's not a valid region!
Conclusion
I hope this tutorial was helpful. This method of using regions is very versatile and could be used for other purposes besides fishing as well! If the situation calls for a system that requires an item to be used, and needing to check the tile right in front of the player (maybe a mystery game?), this method may do the trick!
Naturally, further optimizations can be done with this system. This tutorial aimed to create a baseline that is also easy to follow for novices.
Feel free to let me know if you have any feedback or tutorial requests for systems / puzzles!